- create an algorithm for trading based on 1% value swings
Here’s a basic algorithm for trading based on 1% value swings:
- Define your target security: Select a security that you wish to trade based on 1% value swings.
- Set your trading parameters: Define the parameters for your trades, including the amount of capital you’re willing to invest, your desired profit margin, and your stop-loss limit.
- Monitor the security’s value: Continuously monitor the value of your target security. You can use various tools such as trading software, financial news sources, or chart analysis to track its value.
- Identify 1% value swings: When the security’s value changes by 1% in either direction, take note of it.
- Buy or sell: If the value of the security has decreased by 1%, and you believe it will rebound, consider buying the security. Conversely, if the value of the security has increased by 1%, and you believe it may drop, consider selling the security.
- Set stop-loss limit: To manage risk, set a stop-loss limit for each trade. This is the maximum amount of money you’re willing to lose if the trade doesn’t go in your favor.
- Take profit: Set a profit target for each trade, which is the desired amount of profit you want to earn. When the security’s value reaches this target, close the trade and take your profit.
- Repeat the process: Continue monitoring the security’s value, identifying 1% swings, and making trades as necessary.
Note: This is a basic algorithm, and there are many factors to consider when trading, such as market conditions, liquidity, volatility, and more. It’s important to do your research and fully understand the risks involved before implementing any trading strategy.
2. write this is pseudocode
Sure, here’s the pseudocode for the trading algorithm based on 1% value swings:
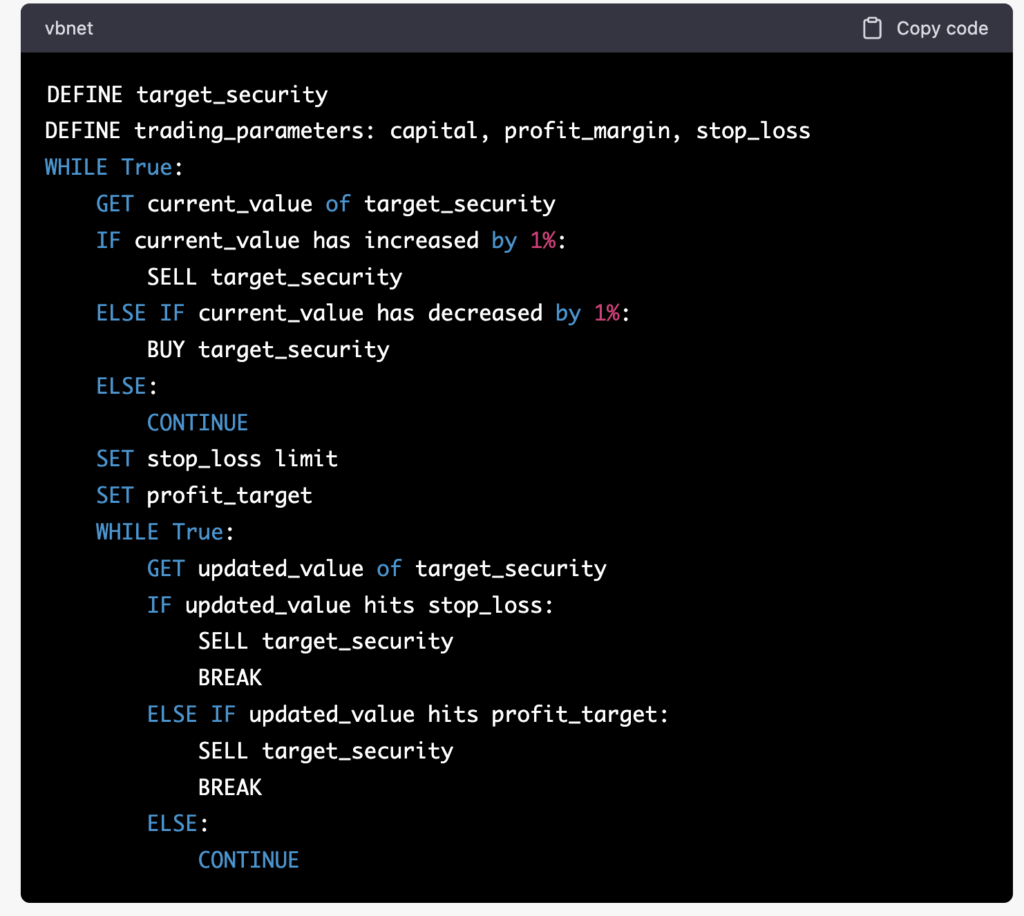
Note that this is a basic pseudocode and it does not include error handling or other advanced features. Additionally, some variables and functions may need to be defined and implemented separately.
3. write this in python
Sure, here’s an example implementation of the trading algorithm based on 1% value swings in Python:
target_security = "AAPL" # define the target security # define the trading parameters capital = 1000 profit_margin = 1.01 # 1% profit margin stop_loss = 0.99 # 1% stop-loss limit while True: current_value = get_current_value(target_security) # get the current value of the target security if current_value >= profit_margin: sell(target_security) # sell the security if it has increased by 1% elif current_value <= stop_loss: buy(target_security) # buy the security if it has decreased by 1% else: continue # otherwise, continue monitoring the value # set stop-loss limit and profit target stop_loss_value = current_value * stop_loss profit_target_value = current_value * profit_margin while True: updated_value = get_current_value(target_security) # get the updated value of the target security if updated_value <= stop_loss_value: sell(target_security) # sell the security if it hits the stop-loss limit break elif updated_value >= profit_target_value: sell(target_security) # sell the security if it hits the profit target break else: continue # otherwise, continue monitoring the value
Note that this is just an example implementation and may need to be adapted to your specific trading platform or strategy. Also, the get_current_value
, buy
, and sell
functions are not defined here and need to be implemented separately based on the API or service you’re using.
And now for some fun
write this for a quantum computer
While quantum computers have the potential to solve certain financial problems more efficiently than classical computers, it’s currently unclear how exactly they could be used for trading based on 1% value swings. As quantum computing is still in its early stages of development, there are few, if any, quantum trading algorithms that have been developed and tested at scale.
However, one possible approach to leveraging quantum computing for trading is by using quantum machine learning algorithms to analyze financial data and identify patterns that can be used to make trading decisions. For example, one could use quantum annealing to optimize portfolio selection and risk management, or quantum algorithms to perform efficient optimization of trading strategies.
Here’s an example of how one could use the Qiskit framework in Python to implement a simple quantum machine learning algorithm for financial prediction:
import numpy as np from qiskit import Aer from qiskit.circuit.library import ZZFeatureMap from qiskit.aqua.algorithms import VQC from qiskit.aqua.components.optimizers import COBYLA # Define the target security and trading parameters target_security = "AAPL" capital = 1000 profit_margin = 1.01 stop_loss = 0.99 # Define the quantum feature map and backend feature_map = ZZFeatureMap(feature_dimension=2) backend = Aer.get_backend('qasm_simulator') # Define the VQC classifier and optimizer classifier = VQC(feature_map, None, None) optimizer = COBYLA() # Train the classifier on historical financial data data = np.array([[0.5, 0.1], [0.1, 0.9], [0.2, 0.8], [0.3, 0.7], [0.4, 0.6]]) labels = np.array([0, 1, 1, 0, 0]) classifier.train(data, labels) # Use the classifier to make trading decisions while True: current_data = np.array([[get_current_price(target_security), get_current_volume(target_security)]]) predicted_label = classifier.predict(current_data)[0] if predicted_label == 0: buy(target_security) elif predicted_label == 1: sell(target_security) else: continue # Set the stop-loss limit and profit target stop_loss_value = get_current_price(target_security) * stop_loss profit_target_value = get_current_price(target_security) * profit_margin while True: updated_price = get_current_price(target_security) if updated_price <= stop_loss_value: sell(target_security) break elif updated_price >= profit_target_value: sell(target_security) break else: continue
Again, note that this is just a simple example of how one could use quantum machine learning for trading, and many details would need to be filled in depending on the specific application and data available.
So, there we have it. I can now learn how to write code for a quantum computer that might have some bearing on financial insights, with zero knowledge.